Understanding Inheritance in Java
- Bhakti Panchal
- Aug 1, 2023
- 2 min read
Introduction
In object-oriented programming, inheritance is a powerful concept that allows a class (subclass or child class) to inherit properties and behaviors from another class (superclass or parent class). This promotes code reusability and helps in building hierarchies of classes. In Java, inheritance is achieved using the extends keyword. Let's explore the basics of inheritance and its various aspects in Java.
Basic Syntax
The basic syntax of inheritance in Java involves using the extends keyword to establish a relationship between a subclass and a superclass. The subclass inherits all non-private members (fields and methods) from its superclass. Here's an example:
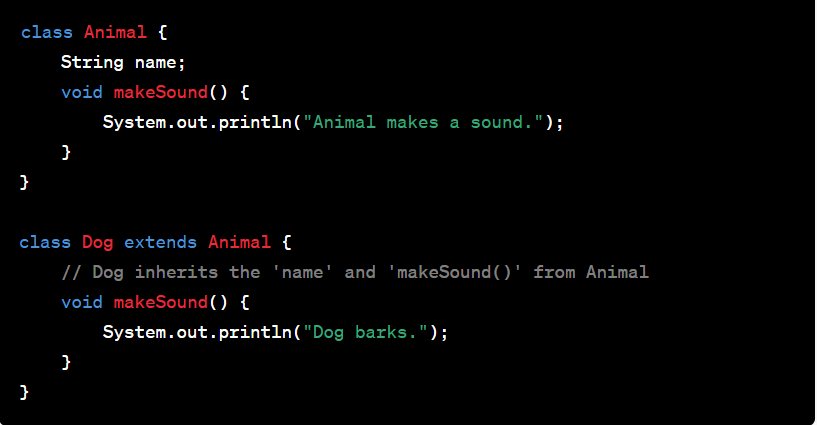
In this example, 'Dog' is a subclass that extends the 'Animal' superclass. The 'Dog' class inherits the 'name' field and the 'makeSound()' method from 'Animal'.
Single Inheritance
Java supports single inheritance, which means a class can have only one direct superclass. In other words, a subclass can extend only one class. For example:
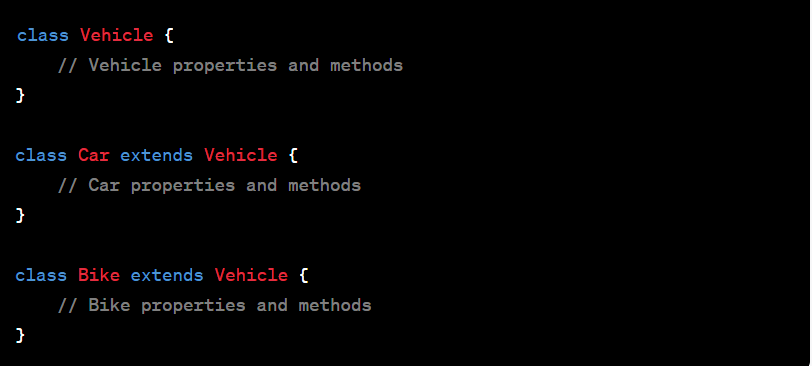
n this example, both 'Car' and 'Bike' are subclasses of Vehicle, which is their common superclass.
Multiple Inheritance (Interface)
Although Java supports single inheritance for classes, it allows multiple inheritance through interfaces. An interface is like a contract that a class can implement to gain certain functionalities. Unlike classes, a class can implement multiple interfaces. Here's an example:
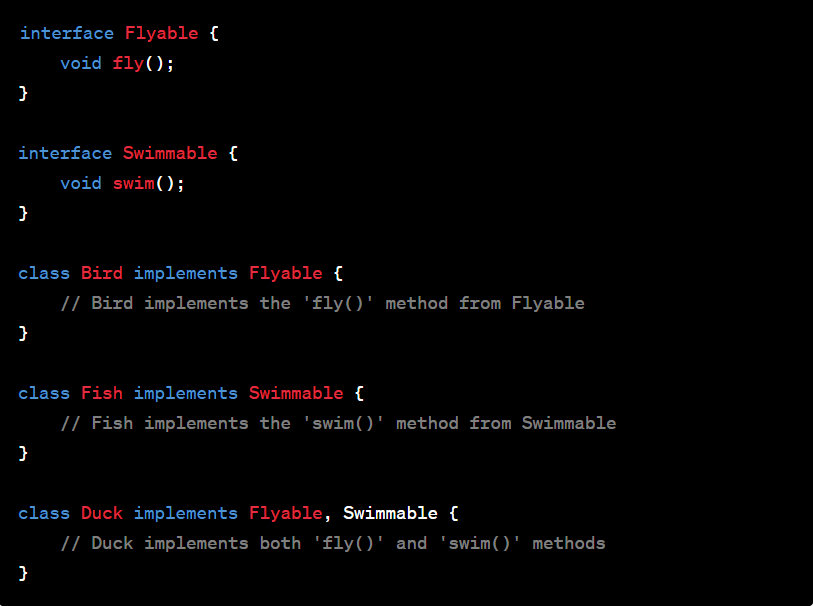
In this example, 'Bird' and 'Fish' implement the 'Flyable' and 'Swimmable' interfaces, respectively. 'Duck' implements both 'Flyable' and 'Swimmable'.
Access Modifiers in Inheritance
Java's access modifiers (public, private, protected, and default) play a role in inheritance. Members with 'public' and 'protected' access are inherited by subclasses, while 'private' members are not inherited. Default access members are inherited if the subclass is in the same package as the superclass. Here's an example:
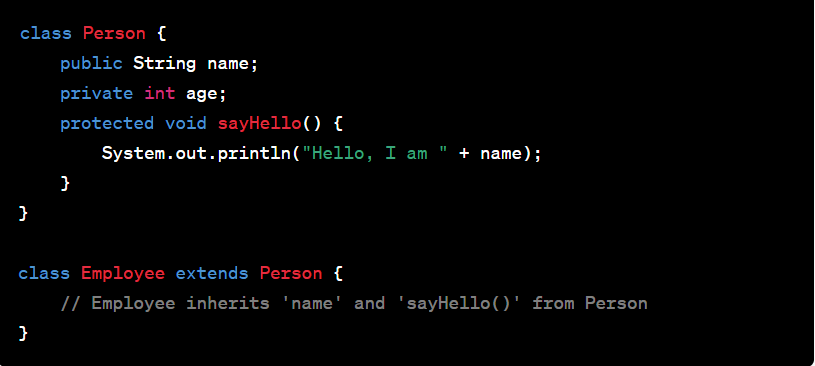
In this example, the 'name' field and the 'sayHello()' method are inherited by the 'Employee' subclass, but the 'age' field (private) is not inherited.
Method Overriding
Method overriding is a crucial feature of inheritance in Java. It allows a subclass to provide its own implementation for a method that is already defined in its superclass. To override a method, the method signature (name and parameters) must be the same in the subclass and the superclass. Here's an example:
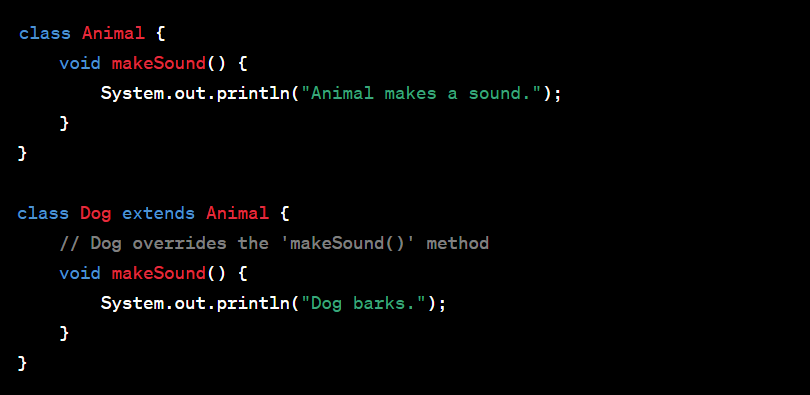
In this example, the 'Dog' class overrides the 'makeSound()' method defined in the 'Animal' class.
Conclusion
Inheritance is a fundamental concept in Java that facilitates code reusability and object-oriented design. It enables the creation of class hierarchies, polymorphism, and abstract data types. By understanding inheritance and its various aspects, Java developers can write more efficient and maintainable code.
In this blog post, we covered the basics of inheritance, including single inheritance, multiple inheritance through interfaces, access modifiers, method overriding, and more. As you progress in your Java journey, mastering inheritance will be invaluable in building robust and scalable applications.
Comentarios