The Six Pillars of Object-Oriented Programming in Java
- ankurpatel2608
- Oct 10, 2023
- 2 min read
Introduction
Object-Oriented Programming (OOP) is a fundamental paradigm in software development, and Java is one of the most popular languages that embraces it. In Java, OOP is based on six key principles, often referred to as the "Six Pillars of OOP." These principles provide a solid foundation for designing, organizing, and maintaining Java applications. In this blog post, we will explore each of these pillars and understand how they contribute to the robustness and maintainability of Java code.
1. Encapsulation
Encapsulation is the concept of bundling data (attributes) and methods (functions) that operate on that data into a single unit known as a class. It's like putting data and the operations on that data in a protective capsule. In Java, classes serve as the blueprint for objects, and they encapsulate both state (fields) and behavior (methods). Access to the internal state is controlled through access modifiers like private, protected, and public, allowing for data hiding and preventing unauthorized access or modification.
Example:
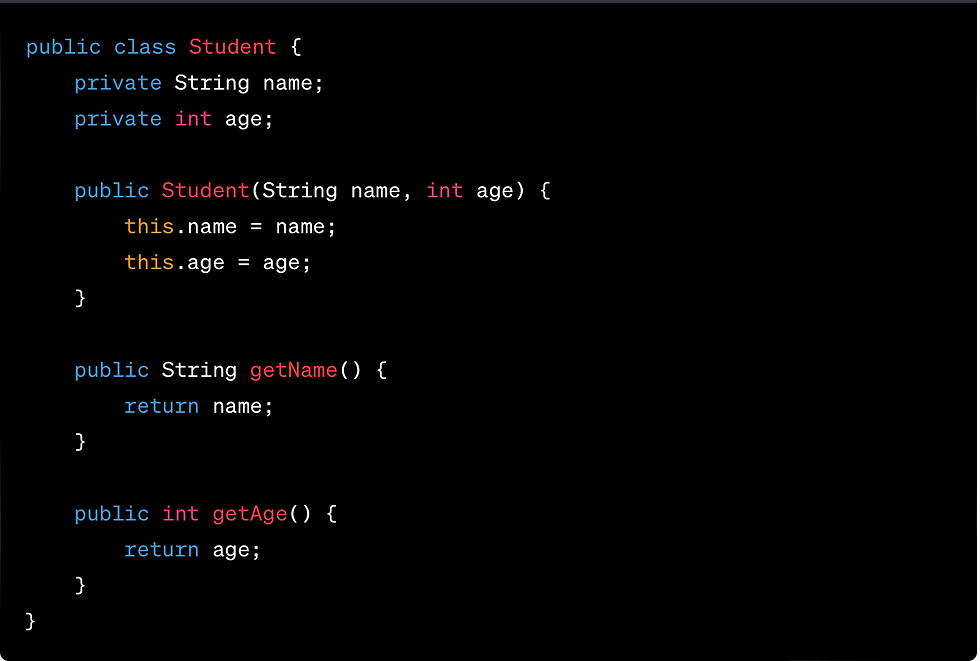
2. Inheritance
Inheritance is a mechanism that allows a class to inherit properties and methods from another class, creating a hierarchical relationship between classes. In Java, a subclass can inherit attributes and methods from a superclass, promoting code reusability. The extends keyword is used to establish inheritance.
Example:

3. Abstraction
Abstraction is the process of simplifying complex systems by breaking them into smaller, more manageable parts. In Java, abstract classes and interfaces are used to achieve abstraction. An abstract class can have abstract methods (methods without a body) that must be implemented by concrete subclasses. Interfaces define a contract that classes must adhere to by implementing all its methods.
Example:
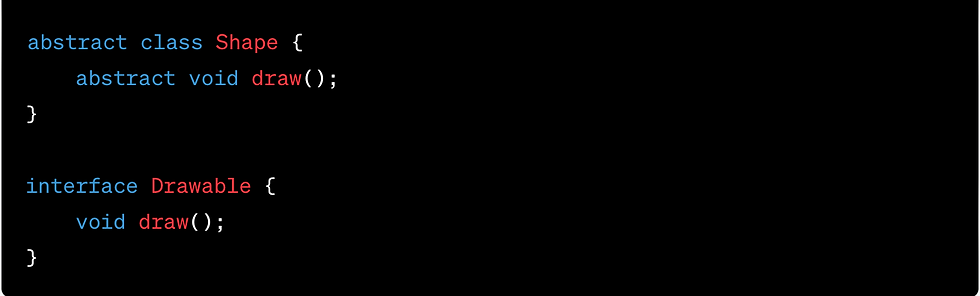
4. Polymorphism
Polymorphism means "many shapes" and is the ability of different classes to be treated as instances of a common superclass. In Java, polymorphism is achieved through method overriding and interfaces. It allows you to write code that works with objects of different classes in a uniform way.
Example:

5. Composition
Composition is a principle where complex objects are created by combining simpler objects. It promotes code reuse and flexibility. In Java, you can achieve composition by including instances of other classes within your class.
Example:
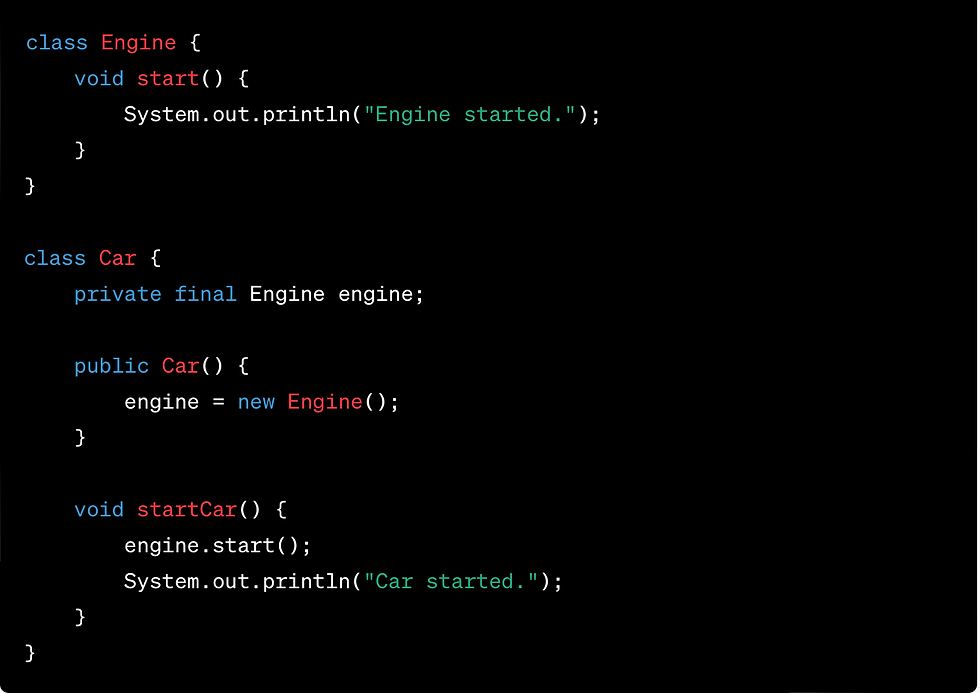
6. Association
Association represents the relationship between two or more classes, emphasizing how objects interact with each other. In Java, associations can be one-to-one, one-to-many, or many-to-many. They are fundamental for building complex systems where objects collaborate to achieve a common goal.
Example:
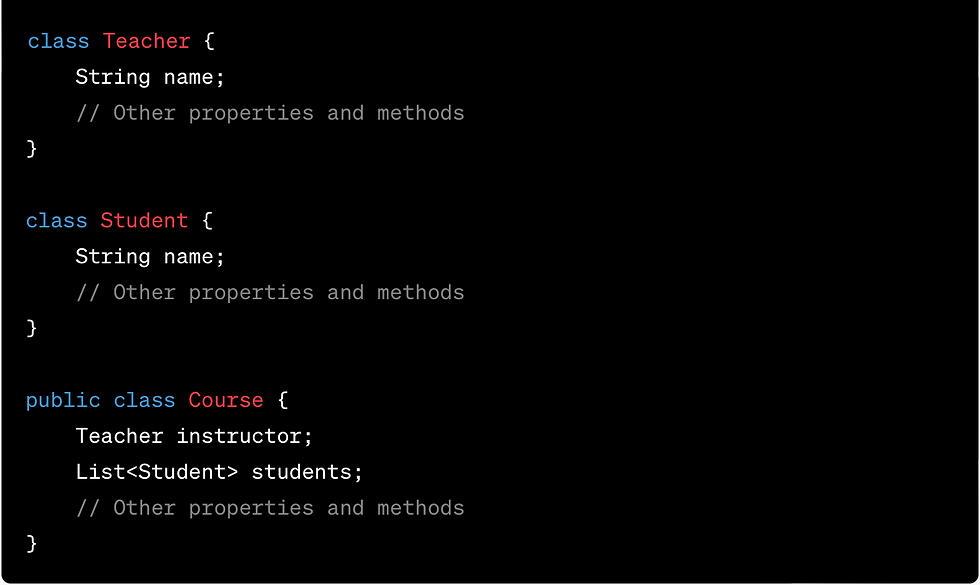
Conclusion
The Six Pillars of OOP in Java provide a comprehensive framework for designing robust and maintainable software. By understanding and applying these principles, Java developers can create modular, reusable, and flexible code, ultimately leading to more efficient development and easier maintenance of software projects. Whether you are building small applications or large-scale systems, OOP principles play a crucial role in structuring your Java code effectively.
Comments