DeepDive into Java Collections
- Urvi Patel
- Dec 11, 2023
- 6 min read
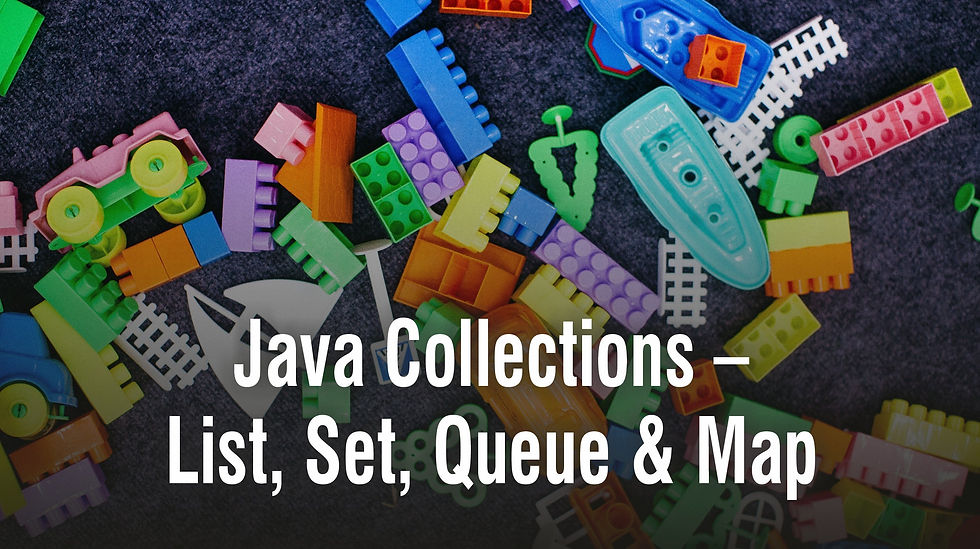
The collection is one of java framework which contains an interface named an iterable interface which provides the iterator to iterate through all the collections. Java Collections Framework interfaces provides the abstract data type to represent collection. The collection framework represents a unified architecture for storing and manupulationg a group of objects.
Hierarchy of Collection Framework
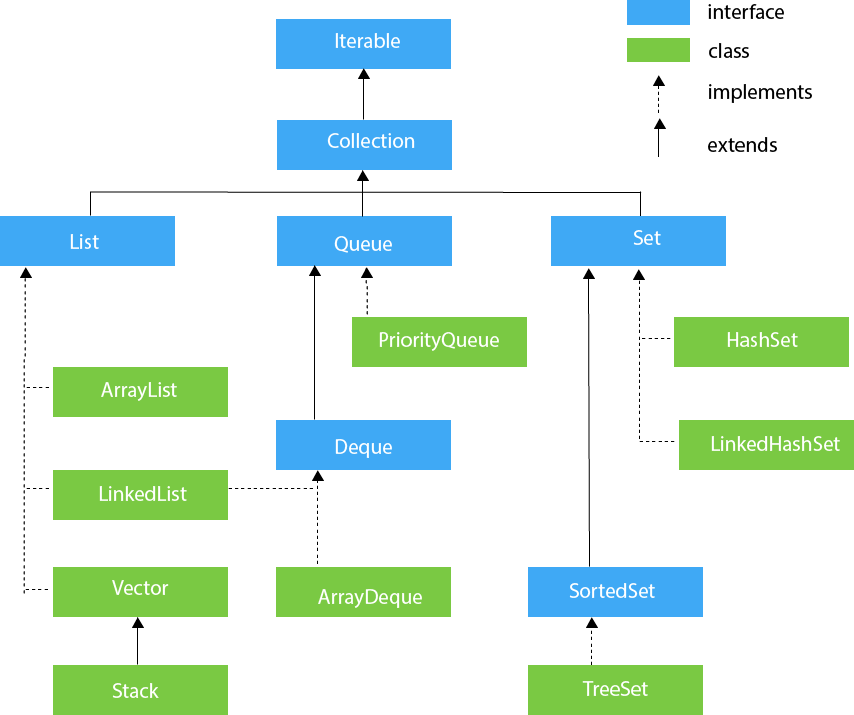
Iterator and Iterable are interfaces that provide a way to iterate over a collection of elements. Let's first understand a class and an interface.
Class: A class is a user-defined blueprint or prototype from which objects are created. It represents the set of properties or methods that are common to all objects of one type.
Interface: Like a class, an interface can have methods and variables, but the methods declared in an interface are by default abstract. Interfaces specify what a class must do and not how. It is the blueprint of the class.
Advantages of the Collection Framework
Reduce Programming Effort - By providing useful data structures and algorithms, the Collections Framework frees you to concentrate on the important parts of your program rather than on the low-level "plumbing" required to make it work. By facilitating interoperability among unrelated APIs, the Java Collections Framework frees you from writing adapter objects or conversion code to connect APIs.
Increase Program Speed and Quality - This Collections Framework provides high-performance, high-quality implementations of useful data structures and algorithms. The various implementations of each interface are interchangeable, so programs can be easily tuned by switching collection implementations. Because you're freed from the drudgery of writing your own data structures, you'll have more time to devote to improving programs' quality and performance.
Better Quality – Using core collection classes that are well-tested increases our program quality rather than using any home-developed data structure.
Reusability and Interoperability - Java Collections provide reusable data structures that can be easily integrated into different applications. The uniform interfaces make it simple to switch between implementations without altering the application's core logic.
Collections API Interfaces
Iterable Interface: This is the root interface for the entire collection framework. The collection interface extends the iterable interface. Therefore, inherently, all the interfaces and classes implement this interface. The main functionality of this interface is to provide an iterator for the collections. Therefore, this interface contains only one abstract method which is the iterator.
Collection Interface: A collection represents a group of objects known as its elements. The Java platform doesn’t provide any direct implementations of this interface.Collection interface also provides different operations methods that work on the entire collection which are containsAll, addAll, removeAll, retainAll, clear.
List Interface: List is an ordered collection and can contain duplicate elements. You can access any element from its index. List is more like array with dynamic length. List is one of the most used Collection type. List are classified into the following: 1. ArrayList : ArrayList provides us with dynamic arrays in Java. Though, it may be slower than standard arrays but can be helpful in programs where lots of manipulation in the array is needed. The size of an ArrayList is increased automatically if the collection grows or shrinks if the objects are removed from the collection. Let's see the example. Class DemoArrayList{ public static void main(String args[]) { ArrayList<String> list = new ArrayList <String> (); // creating arraylist // Adding values in ArrayList list.add("James"); list.add("Robin"); list.add("Nick"); list.add("Robin"); // Traversing list through iterator Iterator itr = list.iterator(); while(itr.hasNext()) { System.out.println(itr.next()); } } } Output: James Robin Nick Robin 2. LinkedList : Linked List is a sequence of links which contains items. Each link contains a connection to another link.The elements are linked using pointers and addresses and each element is known as a node. It can store the duplicate elements. It maintains the insertion order and is not synchronized. In LinkedList, the manipulation is fast because no shifting is required. Let's see the example. public class DemoLinkedList { public static void main(String args[]) { LinkedList<String> linkedlist = new LinkedList<String>(); linkedlist.add("Jason"); linkedlist .add("Samantha"); linkedlist.add("Jason"); linkedlist.add("Mike"); Iterator<String> itr= linkedlist.iterator(); while(itr.hasNext()){ System.out.println(itr.next()); } } } Output: Jason Samantha Jason Mike 3. Vector : A vector provides us with dynamic arrays in Java. Though, it may be slower than standard arrays but can be helpful in programs where lots of manipulation in the array is needed.However, the primary difference between a vector and an ArrayList is that a Vector is synchronized and an ArrayList is non-synchronized. Let's see the example. public Class DemoVector { public static void main(String args[]) { Vector<Integer> v = new Vector<Integer>(4); v.add(3); v.add(8); v.add(5); v.add(1); System.out.println(“Values is vector : ” +v); v.add(9); System.out.println(“Values in vector : ” +v); } } Output: Values in vector : [3, 8, 5, 1] Values in vector : [3, 8, 5, 1, 9] 4. Stack : It is a subclass of vector and implements Last-In-First-Out data structure. The stack contains all of the methods of Vector class and also provides its methods like boolean push(), boolean peek(), boolean push(object o), which defines its properties.Let's see the example. Class DemoStack { public static void main(String args[]) { Stack<String> st = new Stack<>(); // Adding elements st.push(“Dog”); st.push(“Cat”); st.push(“Horse”); st.push(“Rabbit”); System.out.println(“Values in stack: ” +st); //Removing the elements String element = st.pop(); System.out.println(“Removed Element:” +element); System.out.println(“Values in stack after removing the element: ” +st); } } Output: Values in stack: [Dog, Cat, Horse, Rabbit] Removed Element: Rabbit Values in stack after removing the element: [Dog, Cat, Horse]
Queue Interface: Queue is a collection used to hold multiple elements prior to processing. Besides basic Collection operations, a Queue provides additional insertion, extraction, and inspection operations. It maintains the FIFO (First-In-First-Out) order. This interface is dedicated to storing all the elements where the order of the elements matter. For example, whenever we try to book a ticket, the tickets are sold on a first come first serve basis. Therefore, the person whose request arrives first into the queue gets the ticket. PriorityQueue : This class implements the queue interface. It does not allow null values to be stored in the queue. PriorityQueue interface used when the objects are supposed to processed based on thier priority. Lets see the example. public class DemoPriorityQueue { public static void main(String args[]) { PriorityQueue<Integer> n = new PriorityQueue<>(); //Adding the values n.add(7); n.add(4); System.out.println(“PriorityQueue:” +n); //updating the queue with offer() method n.offer(2); System.out.println(“Updated PriorityQueue: ” +n); } } Output: PriorityQueue: [4,7] Updated PriorityQueue: [2,4,7]
Deque Interface: This interface supports element insertion and removal at both ends. The name deque is short for “double-ended queue” and is usually pronounced “deck”. ArrayDeque is faster than ArrayList and Stack and has no capacity restrictions. ArrayDeque : This is a special kind of array that allows user to add and remove elements from both side of the queue.Array deques have no capacity restrictions and they grow as necessary to support usage. ArrayDeque is fasther than ArrayList and Stack and has no capacity restrictions.Lets see the example. public class DemoArrayDeque { public static void main(String[] args) { Deque<String> adeque=new ArrayDeque<String>(); adeque.offer("Ben"); adeque.offer("Kevin"); aadeque.add("Daniel"); adeque.offerFirst("Travis"); System.out.println("After offerFirst Traversal : "); for(String s : adeque) { System.out.println(s); } //adeque.poll(); //adeque.pollFirst();//it is same as poll() adeque.pollLast(); System.out.println("After poll Traversal : "); for(String s : adeque) { System.out.println(s); } } } Output: After offerFirst Traversal : Travis Ben Kevin Daniel After poll Traversal : Ben Kevin Daniel
Set Interface: A set is an unordered collection of objects in which duplicate values cannot be stored. This collection is used when we wish to avoid the duplication of the objects and wish to store only the unique objects. This set interface is implemented by various classes like HashSet, TreeSet, LinkedHashSet, etc. HashSet : The class is an inherent implementation of the hash table data structure. The objects which we insert into the HashSet do not guarantee to be inserted in the same order. The objects are inserted based on their hashcode. This class allows NULL elements. See the example. public class DemoHashSet { public static void main(String args[]) { //creating Hashset and adding values HashSet<String> set = new HashSet<String>(); set.add(“Ron”); set.add(“John”); set.add(“Ron”); set.add(“Brian”); //Traversing the values Iterator<String> itr = set.iterator(); while(itr.hasNext()) { System.out.println(itr.next()); } } } Output: John Ron Brian LinkedHash Set : This set is similar to hashset. The only difference between both is that this uses a double linked list to store data and retains the ordering of the elements. See the example. public class DemoLinkedHashSet { public static void main(String args[]) { LinkedHashSet<String> linkset = new LinkedHashSet<String>(); linkset.add(“Ron”); linkset add(“John”); linkset.add(“Ron”); linkset.add(“Brian”); //Traversing the values Iterator<String> itr = linkset.iterator(); while(itr.hasNext()) { System.out.println(itr.next()); } } } Output: Ron John Brian
SortedSet Interface: This interface is very similar to the set interface. The only difference is that this interface has extra methods that maintain the ordering of the elements. The sorted set interface extends the set interface and is used to handle the data which needs to be sorted. The class which implements this interface is TreeSet. TreeSet : TreeSet class implements the Set interface that uses a tree for storage. The objects of this class are stored in the ascending order. It only contains unique elements.However, the access and retrieval time of TreeSet is quite fast. The elements in TreeSet stored in ascending order.
In conclusion, Java Collections play a vital role in managing and manipulating groups of objects, providing a powerful and flexible framework. Whether dealing with lists, sets, maps, or queues, the Java Collections Framework offers a comprehensive suite of interfaces and classes that simplify the process of handling data structures.
Commentaires